mirror of
https://github.com/khuedoan/homelab.git
synced 2024-12-23 01:04:32 +07:00
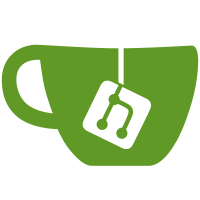
Replaced by Woodpecker CI. It turns out I don't need that much power from Tekton's flexibility, so it's not worth the maintenance overhead for my specific use case at home.
57 lines
1.5 KiB
Python
Executable File
57 lines
1.5 KiB
Python
Executable File
#!/usr/bin/env python
|
|
|
|
# WIP
|
|
# - [x] take screenshot
|
|
# - [ ] self contained
|
|
# - [ ] login automatically credentials from Kubernetes Secrets (is this really needed?)
|
|
|
|
# TODO put this in tools container or use Docker
|
|
# pip install selenium
|
|
# sudo pacman -S geckodriver
|
|
|
|
import time
|
|
from selenium import webdriver
|
|
|
|
apps = [
|
|
{
|
|
'name': 'home',
|
|
'url': 'https://home.khuedoan.com'
|
|
},
|
|
{
|
|
'name': 'gitea',
|
|
'url': 'https://git.khuedoan.com/ops/homelab'
|
|
},
|
|
{
|
|
'name': 'argocd',
|
|
'url': 'https://argocd.khuedoan.com/applications/root'
|
|
},
|
|
{
|
|
'name': 'matrix',
|
|
'url': 'https://chat.khuedoan.com/#/room/#random:matrix.khuedoan.com'
|
|
},
|
|
{
|
|
'name': 'grafana',
|
|
'url': 'https://grafana.khuedoan.com/d/efa86fd1d0c121a26444b636a3f509a8/kubernetes-compute-resources-cluster' # wtf is this ID
|
|
},
|
|
]
|
|
|
|
options = webdriver.firefox.options.Options()
|
|
options.headless = True
|
|
|
|
driver = webdriver.Firefox(
|
|
options=options,
|
|
firefox_profile=webdriver.FirefoxProfile( # TODO deprecated
|
|
profile_directory="/home/khuedoan/.mozilla/firefox/h05irklw.default-release" # TODO do not hard code
|
|
)
|
|
)
|
|
driver.set_window_size(1920, 1080)
|
|
|
|
for app in apps:
|
|
print(f"Opening {app['url']}")
|
|
driver.get(app['url'])
|
|
time.sleep(3) # TODO wait for full page load instead of sleep
|
|
driver.save_screenshot(f"{app['name']}.png")
|
|
print(f"Screenshot saved to {app['name']}.png")
|
|
|
|
driver.close()
|