mirror of
https://github.com/khuedoan/homelab.git
synced 2025-01-05 13:08:52 +07:00
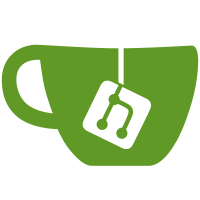
Replaced by Woodpecker CI. It turns out I don't need that much power from Tekton's flexibility, so it's not worth the maintenance overhead for my specific use case at home.
60 lines
1.5 KiB
Go
60 lines
1.5 KiB
Go
package test
|
|
|
|
import (
|
|
"crypto/tls"
|
|
"fmt"
|
|
"testing"
|
|
"time"
|
|
|
|
http_helper "github.com/gruntwork-io/terratest/modules/http-helper"
|
|
"github.com/gruntwork-io/terratest/modules/k8s"
|
|
)
|
|
|
|
func TestSmoke(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
var mainApps = []struct {
|
|
name string
|
|
namespace string
|
|
}{
|
|
{"argocd-server", "argocd"},
|
|
{"gitea", "gitea"},
|
|
{"grafana", "grafana"},
|
|
{"hajimari", "hajimari"},
|
|
{"kanidm", "kanidm"},
|
|
{"registry-docker-registry", "registry"},
|
|
}
|
|
|
|
for _, app := range mainApps {
|
|
app := app // https://github.com/golang/go/wiki/CommonMistakes#using-goroutines-on-loop-iterator-variables
|
|
t.Run(app.name, func(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
options := k8s.NewKubectlOptions("", "", app.namespace)
|
|
|
|
// Wait the service to become available to ensure that we can access it
|
|
k8s.WaitUntilIngressAvailable(t, options, app.name, 30, 60*time.Second)
|
|
|
|
// Now we verify that the service will successfully boot and start serving requests
|
|
ingress := k8s.GetIngress(t, options, app.name)
|
|
|
|
// Setup a TLS configuration, ignore the certificate because we may not use cert-manager (like the sandbox environment)
|
|
tlsConfig := tls.Config{
|
|
InsecureSkipVerify: true,
|
|
}
|
|
|
|
// Test the endpoint, this will only fail if we timeout waiting for the service to return a 200 response
|
|
http_helper.HttpGetWithRetryWithCustomValidation(
|
|
t,
|
|
fmt.Sprintf("https://%s", ingress.Spec.Rules[0].Host),
|
|
&tlsConfig,
|
|
30,
|
|
60*time.Second,
|
|
func(statusCode int, body string) bool {
|
|
return statusCode == 200
|
|
},
|
|
)
|
|
})
|
|
}
|
|
}
|